Table of Contents
You might have already heard of such a term as pseudocode, especially if you are a software engineer. Pseudocode enables developers and non-developers to write the logic of code in a clear and free style. In spite of the fact that it does not contain real code, it is more than just a rough sketch of a software project. Pseudocode is a valuable tool to express algorithms and navigate the intricacies of coding independently of any specific programming language syntax.
This is not all.
In this article, we will answer the “What is pseudocode?” question in detail. Moreover, we will discuss how to write it and how it can be helpful for addressing various programming issues.
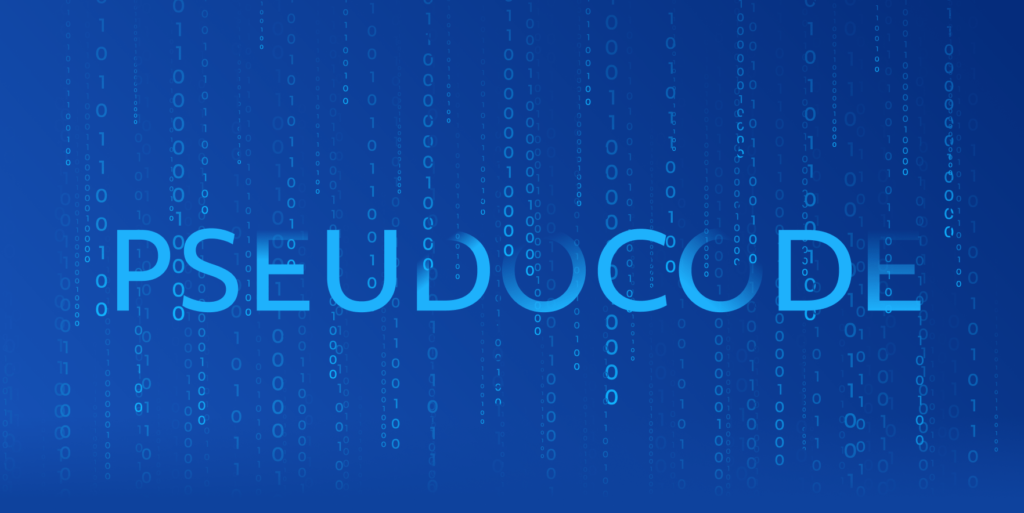
What is pseudocode?
Pseudocode is a versatile concept that extends beyond programming and is used in numerous fields, such as mathematics or design. As the name implies, pseudocode is “fake” code. In other words, it is an informal way of designing the logic and the structure of an application or algorithm. Unlike specific programming languages with rigid syntax rules, pseudocode uses human-readable language to describe logical steps of specific actions. For this reason, it does not have a standard writing format. So, it may vary widely in style from person to person.
Pseudocode consists of essential elements common across various algorithms:
- Variables: represented using simple names to denote data storage;
- Operations: basic operations such as assignment, arithmetic, and conditional statements;
- Loops: described using intuitive language like “while” or “for”;
- Functions/Procedures: represented as named blocks of code with parameters and returns.
Software developers often use it to formulate and build algorithms before the coding stage. Then, to turn it into machine-readable code, they must translate it into their preferred coding language. By creating it first, programmers can focus on the algorithm’s design (as well as how it will function) rather than worrying about the quality of syntax.
Often, developers use both flowcharts and pseudocode in conjunction. While pseudocode is a text-based format that provides a detailed description of the algorithm, flowcharts use a graphical format (diagram) to illustrate the flow of decisions. Thus, developers can plan an algorithm’s logic effectively. So, among other advantages, it promotes a more thought-out approach to programming.
Advantages of pseudocode
It is highly beneficial to design a program in pseudocode for a wide range of reasons, including but not limited to the following:
- Easy to write, understand, and read: pseudocode uses natural language structures and basic concepts, making it easy to read and understand;
- Easy to convert: since it is written in a free style, it can be translated into any coding language;
- Easy to collaborate: unlike scripting language that can be difficult for understanding, it provides a common ground for team members (both tech and non-tech specialists) to work together effectively;
- Easy to change: it is more concise, less complex, and readable than actual code, thus offering a simpler way to incorporate changes before the product is developed;
- Reduces the number of bugs: it allows developers to identify potential bugs before they occur in real programs, thus increasing the speed and quality of the development process;
- Better problem understanding: using pseudocode first, developers can see the logic behind a solution before designing the actual code.
The main challenges of pseudocode
When it comes to the disadvantages of pseudocode, keep in mind the following:
- Lack of a formal standard: pseudocode can differ from one organization to another because there are no standard formats to write it. This can result in inconsistency and ambiguity in its usage, especially if it’s shared between the teams;
- Time-consuming: it can be time-consuming, as the process of translating the pseudocode into actual code may require additional effort and meticulous planning;
- Hard to verify: computers cannot compile or execute pseudocode, so its correctness cannot be verified;
- Complex to map process: due to the lack of visual representation of the process, it can be challenging to convey complicated procedural logic clearly.
In general, pseudocode is a valuable tool that helps the team understand how the final product is supposed to function. And despite the lack of a standard format for pseudocode, there are several universal tips on how to create it – let’s take a look.
How to write pseudocode
As we said above, there is no definite and universal way to write pseudocode due to the lack of a standard format. Although pseudocode can be generated in many ways, it has certain common and structured elements that must be included. They are:
- Sequence: consists of a series of steps performed in a specific order;
- While: a conditional loop that runs until a certain condition is met;
- If-Then-Else: a conditional statement that takes different actions depending on whether it is true or false;
- Repeat-Until: a loop that continues until a specified condition becomes true;
- For: a loop that repeats for a specific number of times;
- Case: the generalization form of If-Then-Else.
Let’s look at a pseudocode example for a better explanation.
A pseudocode example
Before diving into coding, make sure you know what your pseudocode is supposed to accomplish. Let’s say your intended program will allow users to determine whether a number is even or odd. So, pseudocode may look something like this:
1. Input number // User inputs a number
2. Set a remainder = number% 2 // Calculate the remainder when dividing the number by 2
3. If the remainder is equal to 0 // Check if the remainder is zero
1. Output "The number is even"// If true, the number is even
4. Else
1. Output "The number is odd"// If false, the number is odd
Explanation: the first step prompts the user to input a number. The number serves as the initial data for the subsequent calculations. Then, we calculate the remainder by dividing the number by 2. After that, we use an “If-Else” statement to check if the remainder equals 0. If true, the program outputs “The number is even.” If false, the program outputs “The number is odd.” Thus, it shows a simple decision-making process and describes how an even number is determined.
Remember, it was just an example for creating this program; the approaches may differ greatly.
The do’s of writing pseudocode
Pseudocode effectiveness comes from the ability to clearly explain the algorithm logic. So, for clear and readable pseudocode, be sure to follow these tips:
- Start with a description of the purpose of the process;
- Write only one statement per line;
- Focus on logic, not syntax;
- Use clear terminology;
- Keep the proper order of your pseudocode;
- Use standard programming structures, like “if-then” and “while,” else”;
- Organize your pseudocode sections;
- Double-check your pseudocode.
The don’ts of writing pseudocode
To ensure clarity, conciseness, and effectiveness of pseudocode, it is equally important to know pitfalls to prevent when designing pseudocode. Here are a few of the main considerations:
- Avoid extra details that are better for actual code;
- Do not skip indentation;
- Do not use ambiguous or vague terms;
- Do not overcomplicate;
- Do not ignore structure;
- Do not use programming language keywords;
- Do not skip testing.
Now that we better understand the main do’s and don’ts, let’s review the steps that developers may find helpful during coding.
How to solve programming problems by using pseudocode
For developers, pseudocode may simplify the development process. Rather than focusing solely on technical details like coding syntax and structure, developers can prioritize the logical part. Here are some steps that developers can take during pseudocoding:
Step 1. Understand the problem
When it comes to coding, the understanding of the problem is vital (a task you need to accomplish). Pseudocode allows developers to describe their interpretation of the problem in natural language. In other words, developers will identify the problem’s inputs, outputs, and specific conditions before moving forward.
Step 2. Understand the question
If programmers understand the issue(s), they outline the steps necessary to solve the problem and hence comes to a solution. Thus, a clear understanding of all aspects of the issue is the next step towards a solution.
Step.3 Break down the problem
Divide the problem into smaller sub-problems or tasks to allow you to solve it more easily. By solving a small problem, you are getting closer to solving a big one. After solving each step of the problem, check the result to see if you are on the right track. Keep solving these small problems until you find a solution to the problem.
Step.4 Bring in actual code and tools
When the problem is thoroughly explored and broken down into manageable steps, developers can smoothly transition from pseudocode to real coding. Pseudocode serves as a template for implementation, easing the coding process. This way, programmers can choose the most appropriate software constructs, logic flow, and algorithmic solutions. Many websites are available to help with this, such as Mozilla Developer Network, W3Schools, Stack Overflow, and others.
By following these steps, developers can leverage pseudocode as an effective tool to conceptualize, design, and explain programming solutions before diving into more detailed coding procedure.
Conclusion
Pseudocode in programming is not an indispensable solution for solving programming issues across developers. Instead, it is a helpful method for uncovering ambiguous decisions regarding a specific problem in web development, data science, design, and other fields. Therefore, the understanding of “what is pseudocode and how to deal with it” lays the foundation for crafting code that works and is concise.
Expert Opinion
Pseudocode is an excellent way to describe the process without spending much time writing code. Nowadays, there are more options for describing the steps of a program. It is also a good solution to use flowcharts with pseudocode together, if time is not a problem, to help individuals see the code parts more clearly. Aside from that, I would like to emphasize that writing pseudocode can be a good way to train young developers as well.
.NET Developer at SoftTeco
Sergey Ostapuk
Comments